Unlocking the Power of Coding
If you're new to coding, Python is one of the best languages to start with. It’s easy to learn, versatile, and used by companies all over the world. Whether you want to create games, build websites, or analyze data, Python is a great place to begin your coding journey.
Why Python?
Python’s syntax (the way the code is written) is clean and readable, making it perfect for beginners. Plus, it's powerful enough for professionals to use for advanced tasks, like machine learning and artificial intelligence.
To practice Python, open up this link in your favorite web browser: https://www.jdoodle.com
Your First Python Program: "Hello, World!"
Every coder’s journey starts with the iconic "Hello, World!" program. Here’s how you can write it:
print("Hello, World!")
Working with Variables & Loops
Variables are like storage containers for data. You can use them to store numbers, text, or other information. Here's an example of using a variable of a For Loop:
# this is a For loop
x = 0 # the variable x is set to zero
for x in range(10):
print(x)
x+=1
Here's an example of using a variable of a While Loop:
print() #prints a blank line
y = 0 # the variable y is set to zero
while y < 10:
print(y)
y+=1
Conclusion
# import the random module from the Python library
import random
z = random.randint(1,10) # generates a random number between 1 and 10
# The If/Then Statement compares and then evaluates whether the conditionis True or False
if z < 5:
print(z, " is less than 5")
elif z > 5:
print(z," is greater than 5")
else:
print(z, " is equal to 5")
Python is a powerful tool that can help you build all sorts of amazing projects. The best way to get better at it is to practice and experiment with writing your own code. Start small, and soon you'll be creating your own programs!
Happy coding!
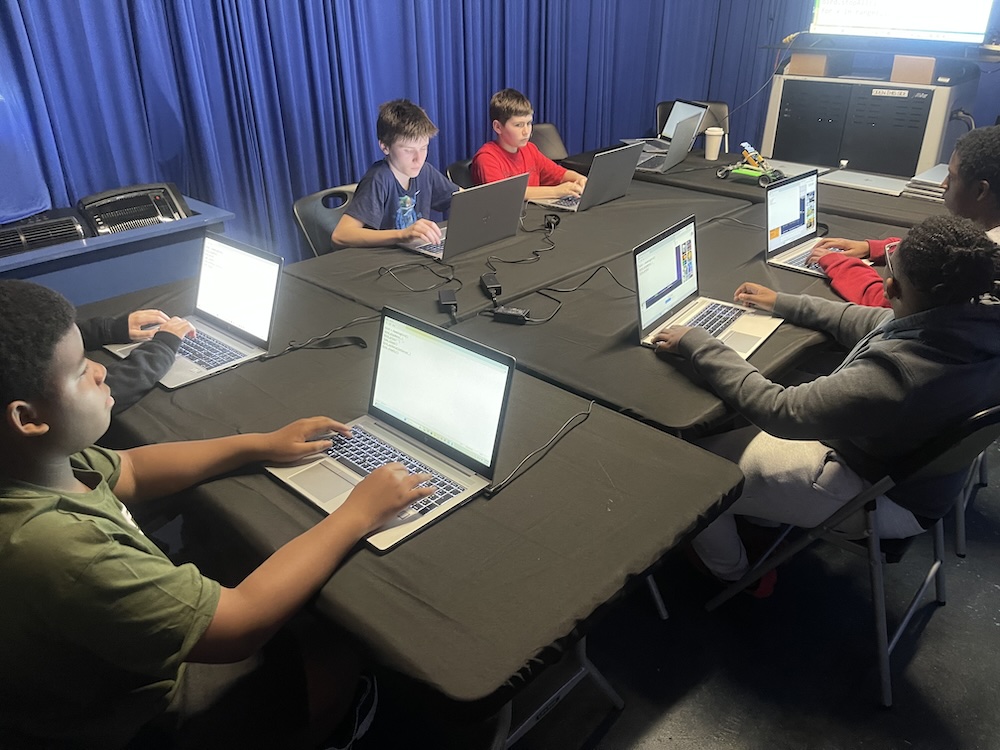