Review:
It's important to continually practice some of the basics of Python until you gain comfort with the syntax. Syntax means how the language is written. Using variables,loops, and print functions are super important. Let's practice these concepts using the For and While loops.
# import the random module from the Python library
import random
# this is a For loop
x = 0 # the variable x is set to zero
for x in range(10):
print(x)
x+=1
print() #prints a blank line
y = 0 # the variable y is set to zero
while y < 10:
print(y)
y+=1
print() #prints a blank line
z = random.randint(1,10) # generates a random number between 1 and 10
# The If/Then Statement compares and then evaluates whether the conditionis True or False
if z < 5:
print(z, " is less than 5")
elif z > 5:
print(z," is greater than 5")
else:
print(z, " is equal to 5")
Our Hummingbird Robotic Car is configured to move forward and reverse, display letters and numbers in the LED display, and play notes. The time.sleep function does not stop activity, but extends the duration of an action for the time indicated in the (). For example: (1) = 1 second.
The code below instructs the car to:
- stop all activity at the beginning as a reset
- play a note
- print 1, 2, 3, on the LED
- set the right and left wheels in motion for 2 seconds
- set the vehicle to turn slightly to the left for 3 seconds
from BirdBrain import Hummingbird, Microbit
import time
bird = Hummingbird()
bird.stopAll()
bird.playNote(60,0.5)
bird.print('1')
time.sleep(1)
bird.print('2')
time.sleep(1)
bird.print('3')
time.sleep(1)
bird.setRotationServo(2, -70)
bird.setRotationServo(3, 20)
time.sleep(2)
bird.setRotationServo(2, -70)
bird.setRotationServo(3, 100)
time.sleep(3)
bird.stopAll()
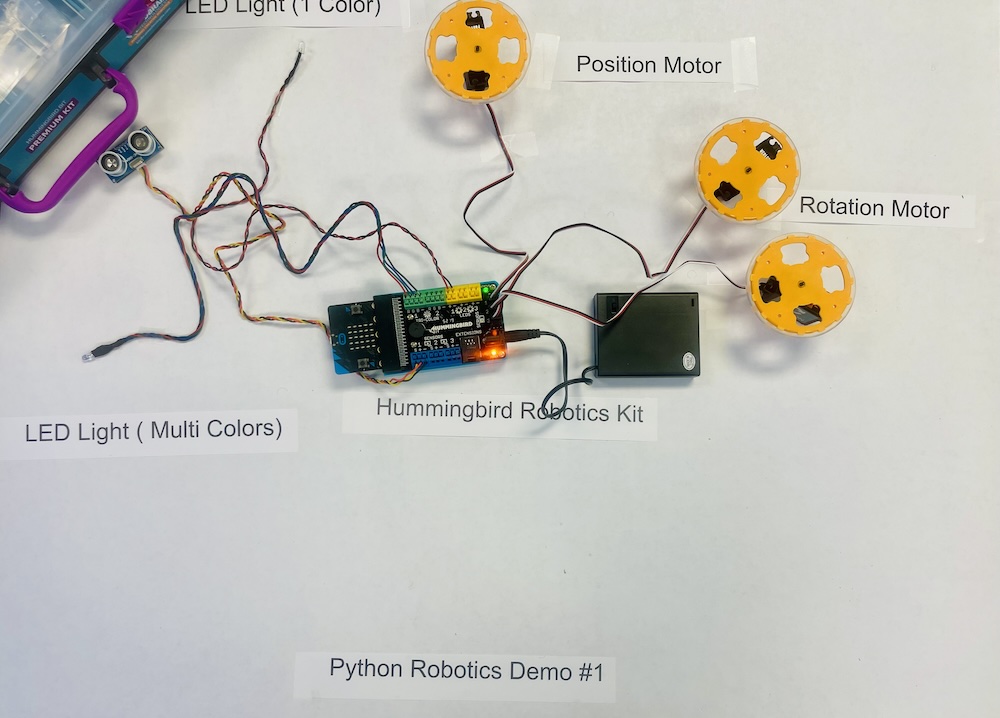