Warm-Up Activity
We're going to use a built-in Python module called Turtle to explore more Python concepts:
import turtle as t
# t.penup()
# t.pendown()
# t.bgcolor()
# t.color()
# t.pensize()
# t.speed()
# t.begin_fill()
# t.end_fill()
# t.fillcolor()
# t.forward()
# t.backward()
# t.left()
# t.right(0)
# t.circle()
# t.setheading()
# t.hideturtle()
# t.home()
# t.goto()
# t.done()
Python Turtle is a module that provides a drawing board, or canvas, allowing users to command a virtual "turtle" to draw shapes and patterns. It's often used for educational purposes to introduce programming concepts in a visual and engaging way. The turtle can be moved forward, backward, left, or right, and its pen can be raised or lowered to control whether it draws while moving. Users can also change the turtle's color, shape, and the background color of the canvas.
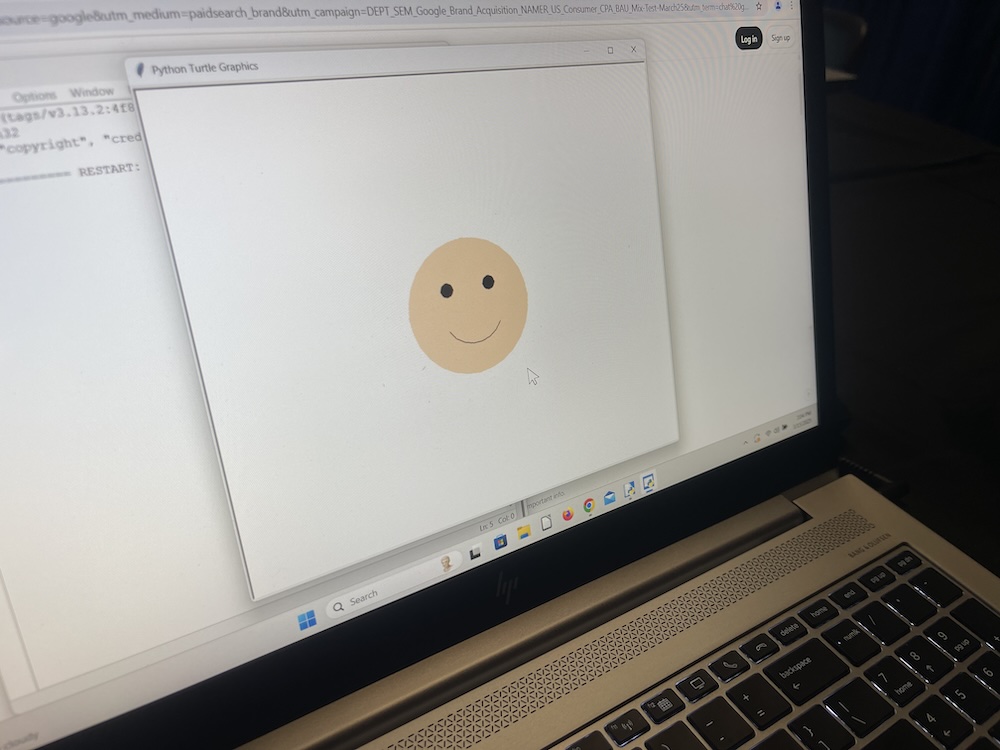
Python Code For Vehicle Operation
This is the starter code for moving the vehicle forward. It's time to drive!
from BirdBrain import Hummingbird, Microbit
import time
myBird = Hummingbird()
myBird.playNote(60,0.5)
myBird.setRotationServo(2, -100)
myBird.setRotationServo(3, 30)
time.sleep(3)
myBird.stopAll()